Greetings to the Readers! In this post, we will see a few examples of Pattern Programming with C language. These patterns are either ‘*’ based or number-based. We hope that students who have C programming as one of their subjects in their graduation (BTech, BCA, BSc, etc.) will find these examples useful.
Drawing Pattern in C language
Q1: Write a C program to draw the pattern in * below.
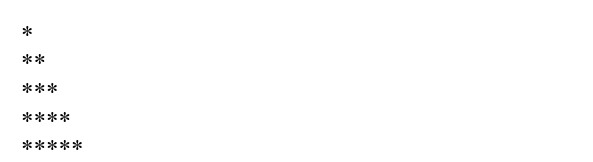
Solution:
#include<stdio.h>
void main()
{
//written by gradguru99.com
int i,j;
for (i=1;i<=5;i++)
{
for(j=1;j<=i;j++)
{
printf(“*”);
}
printf(“\n”);
}
}
Q2: Write a program in C to create the pattern in * below.
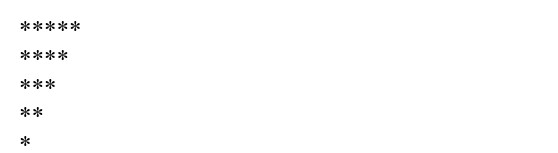
Solution:
#include<stdio.h>
void main()
{
//written by gradguru99.com
int i,j;
for (i=5;i>=1;i–)
{
for(j=i;j>=1;j–)
{
printf(“*”);
}
printf(“\n”);
}
}
Q3: Write a program in C to create the number pattern shown below.
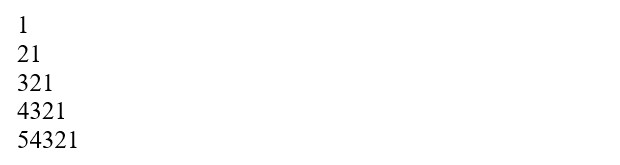
Solution:
#include<stdio.h>
void main()
{
for(int i=1;i<=5;i++)
{
for(int j=i;j>=1;j–)
{
printf(“%d”,j);
}
printf(“\n”);
}
}
Q4: Write a program in C to create the numeric pattern shown below.
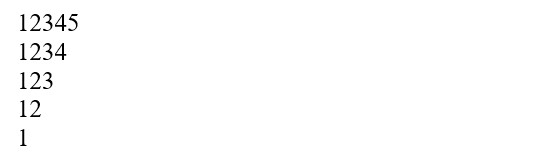
Solution:
#include<stdio.h>
void main()
{
int i,j;for(int i=5;i>=1;i–)
{for(int j=1;j<=i;j++)
{printf(“%d”,j);
}printf(“\n”);
}}